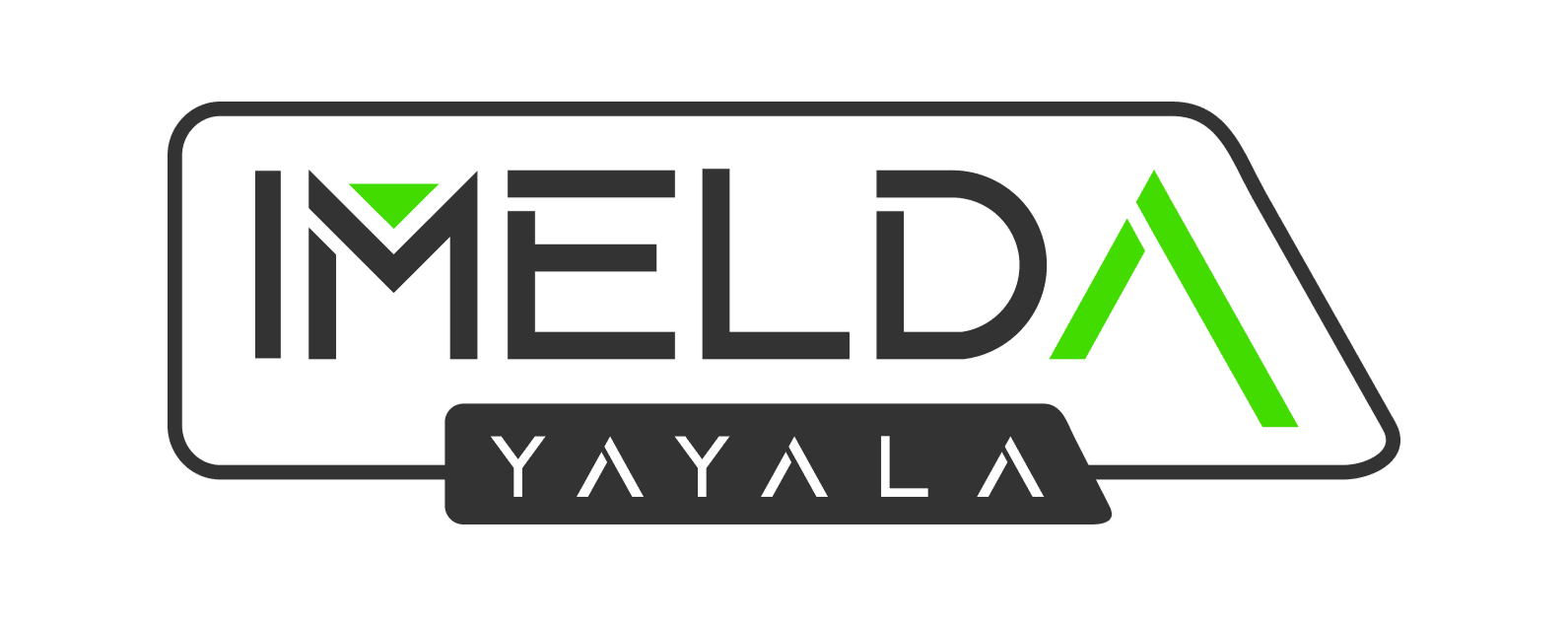
Imelda Yayala: Digital Travel Agency Platform
Imelda Yayala is a traditional travel agency that needed a digital transformation. I developed a comprehensive marketing website with an integrated digital form submission system using React, TypeScript, and Vite. The project streamlined their customer application process, replacing an inefficient manual workflow with a modern, user-friendly online experience.
The platform addresses a critical operational bottleneck where clients previously had to download PDF forms, manually type answers, and submit responses through WhatsApp messages. With the new system, users can complete all required fields directly on the website and submit their applications along with necessary attachments in a single step, significantly improving user experience and operational efficiency.
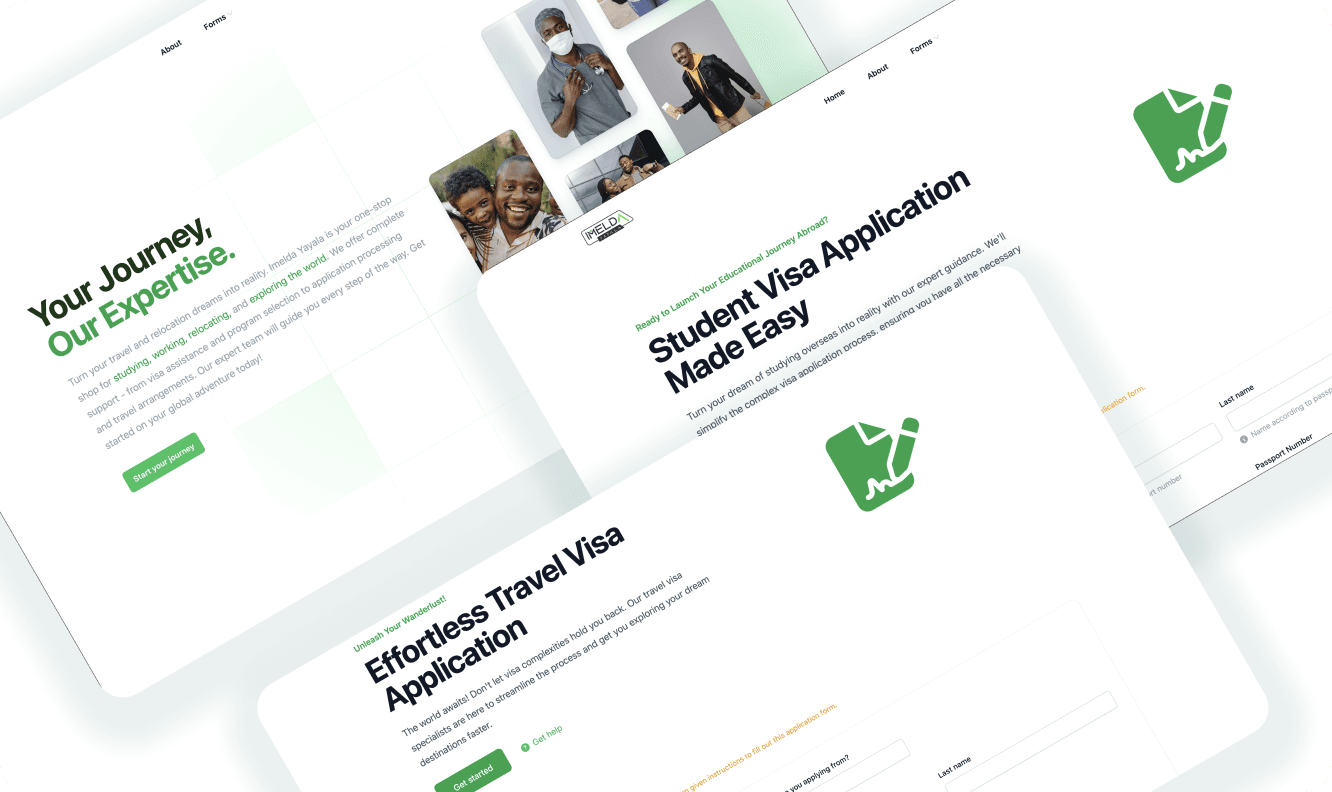
Key Features and Innovations
- Digital Application System: Comprehensive online form that replaced the manual PDF-based process
- Document Upload Functionality: Secure file attachment system for required travel documents
- Email Integration: Automated submission system that delivers completed forms and attachments directly to the agency's email
- Responsive Design: Mobile-friendly interface ensuring accessibility across all devices
- Marketing Website: Professional online presence showcasing the agency's services and offerings
Technical Challenges and Solutions
The project presented several technical challenges that required creative solutions:
Backend Evolution
Initially, I developed a robust backend system with Firebase, but had to pivot based on the client's budget constraints:
// Initial Firebase-based form submission handler
const submitToFirebase = async (values, attachments) => {
try {
// simplified attachment upload
const fileRefs = await Promise.all(
attachments.map((file) => `url/${file.name}`),
)
// simplified document addition
await addDoc(collection(db, 'applications'), {
...values,
attachmentUrls: fileRefs,
})
return { success: true }
} catch (error) {
return { success: false, error: error.message }
}
}
Nodemailer Implementation
To meet budget requirements, I pivoted to a more cost-effective solution using Nodemailer:
// Server-side email handler
app.post('/api/submit-form', upload.array('attachments'), async (req, res) => {
const formData = JSON.parse(req.body.formData)
// Configure email with attachments
const mailOptions = {
from: process.env.EMAIL_FROM,
to: process.env.EMAIL_TO,
subject: `Travel Application: ${formData.fullName}`,
html: generateEmailTemplate(formData),
attachments: req.files.map((file) => ({
filename: file.originalname,
content: file.buffer,
})),
}
// Send email and respond to client
try {
await transporter.sendMail(mailOptions)
res.status(200).json({ success: true })
} catch (error) {
res.status(500).json({ success: false, message: error.message })
}
})
Custom Form Implementation with Formik and Yup
I created a sophisticated form system using Formik with custom input components and Yup for validation:
// Dynamic validation schema with Yup
const validationSchema = Yup.object().shape({
fullName: Yup.string().required('Full name is required'),
destination: Yup.string().required('Destination is required'),
passportNumber: Yup.string().when('travelType', {
is: 'international',
then: Yup.string().required('Passport number is required'),
}),
})
// Custom form input component
const FormField = ({ label, name, ...props }) => {
const [field, meta] = useField(name)
return (
<div>
<label htmlFor={name}>{label}</label>
<input {...field} {...props} />
{meta.touched && meta.error && <div>{meta.error}</div>}
</div>
)
}
File Upload Handling
I implemented a custom file upload component to handle document attachments:
// File upload component
const FileUpload = ({ setFieldValue }) => {
const handleFileChange = (e) => {
setFieldValue('attachments', Array.from(e.target.files))
}
return (
<div>
<input type="file" multiple onChange={handleFileChange} />
</div>
)
}
Collaboration and Client Communication
Working directly with the travel agency required clear communication:
- Initial Requirements Gathering: Conducted detailed interviews to understand their manual process and pain points
- Prototype Development: Created wireframes and mockups to visualize the digital transformation
- Budget Discussions: Openly communicated technical options and helped the client make informed decisions about cost vs. functionality
- User Testing: Involved agency staff in testing the application process to ensure it met their operational needs
User Feedback and Iterative Development
Feedback from the agency and their customers led to several improvements:
"The new online form has cut our application processing time in half. We're spending less time on paperwork and more time helping our clients plan their trips." - Agency Manager
Business Impact
Since launching the platform, Imelda Yayala has experienced:
- 60% reduction in application processing time
- Elimination of data entry errors from manual transcription
- Increased application completion rate due to improved user experience
- Enhanced professional image through modern web presence
Future Roadmap
Potential future enhancements include:
- Customer Portal: Secure login area for clients to track application status
- Payment Integration: Online payment processing for travel services
- Automated Document Verification: System to validate submitted documents
- Analytics Dashboard: Reporting tools to track application metrics and customer demographics
Live Project
Visit the website: Imelda Yayala Website